Introduction
Imagine having an AI model that speaks like your top support agent, analyzes like your smartest analyst, and writes like your best marketer. That is the power of LLM fine-tuning. Off-the-shelf models often miss the mark in real business tasks – but fine-tuning large language models helps build custom LLM models tailored to your needs.
In this blog, you will learn about different LLM fine-tuning approaches, take a technical deep dive into the process, explore top tools and frameworks, understand evaluation techniques, and review deployment steps for production-ready LLMs. We will also cover common pitfalls and how to avoid them – helping you make the most of your AI strategy.
Understanding LLM Fine-Tuning Approaches
LLM fine-tuning is all about customizing pre-trained models to work well for your specific business task. Instead of building a model from scratch, you take a model already trained on massive data (like GPT or FLAN-T5) and then tweak it using your own task-related data. This process makes the model better at handling your exact needs – whether it is summarizing reports, answering customer questions, or processing legal documents.
Let us look at three major approaches to fine-tuning large language models, so you can choose the best one based on your goals and resources.
Full Fine-Tuning – Best Accuracy, High Cost
In full fine-tuning, you adjust all the model’s parameters using labeled training data. This allows the model to learn your task in depth, which is great for custom LLM models that require high accuracy. The steps are simple:
- Load a pre-trained model.
- Feed it with input and expected output.
- Update all parameters using a learning algorithm (backpropagation).
But this method comes at a price. It needs large datasets, powerful GPUs, long training time, and a lot of memory.
For example, fine-tuning an 11-billion parameter model like FLAN-T5 XXL usually requires multiple GPUs and high storage costs.
That is where smarter, lighter alternatives come in.
python
from transformers import AutoModelForSequenceClassification, Trainer, TrainingArguments
# Load pre-trained model
model = AutoModelForSequenceClassification.from_pretrained(
"google-bert/bert-base-cased",
num_labels=5 # For Yelp 5-star classification
)
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
learning_rate=2e-5,
per_device_train_batch_size=16,
num_train_epochs=3,
weight_decay=0.01,
save_strategy="epoch",
)
# Initialize Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_dataset["train"],
eval_dataset=tokenized_dataset["test"],
)
# Start fine-tuning
trainer.train()
Parameter-Efficient Fine-Tuning (PEFT) – Smart, Cost-Saving Choice
Parameter-Efficient Fine-Tuning is a great option when you do not have massive data or infrastructure. Instead of retraining everything, you keep most of the model frozen and update only a small set of parameters.
Here is a quick comparison:
Feature | Full Fine-Tuning | PEFT |
Dataset Size | Large datasets needed | Works with smaller datasets |
Training Speed | Slower | Faster |
Resource Usage | High | Low |
Parameters Updated | All model weights | A small subset |
Overfitting Risk | Higher | Lower |
Among the various PEFT methods, Low-Rank Adaptation (LoRA) is widely used because it works well and is resource-efficient.
LoRA: Low-Rank Adaptation
LoRA modifies a model by inserting compact, trainable matrices alongside its fixed weights, using a technique known as low-rank decomposition. This smart technique greatly reduces the number of parameters that need training while still delivering results close to full fine-tuning.
python
from transformers import AutoModelForCausalLM, AutoTokenizer
from peft import get_peft_model, LoraConfig, TaskType
# Load base model
model = AutoModelForCausalLM.from_pretrained("google/flan-t5-xxl", load_in_8bit=True)
tokenizer = AutoTokenizer.from_pretrained("google/flan-t5-xxl")
# Define LoRA configuration
peft_config = LoraConfig(
task_type=TaskType.CAUSAL_LM,
inference_mode=False,
r=8, # rank of update matrices
lora_alpha=32,
lora_dropout=0.1,
target_modules=["q", "v"] # only apply LoRA to query and value matrices
)
# Create PEFT model
model = get_peft_model(model, peft_config)
# See trainable vs frozen parameters
trainable_params = sum(p.numel() for p in model.parameters() if p.requires_grad)
total_params = sum(p.numel() for p in model.parameters())
print(f"Trainable parameters: {trainable_params} ({trainable_params/total_params:.2%})")
With LoRA, you can fine-tune an 11 billion parameter model like FLAN-T5 XXL on a single GPU, which would be impossible with full fine-tuning.
Instruction Tuning – Teach with Natural Language
Another powerful approach is instruction tuning. Instead of just giving the model examples, you guide it using clear instructions written in natural language. This helps models:
- Adapt faster using less data
- Understand “why” they are doing a task
- Perform better in real conversations
Instruction tuning is gaining popularity in LLM training and tuning because it offers more flexibility and transparency, especially for building conversational agents.
Technical Deep Dive: The Fine-Tuning Process
Get a clear, step-by-step look into how LLM fine-tuning really works – from preparing data to training efficiently with PEFT and LoRA.
Data Preparation and Preprocessing
A strong fine-tuning project begins with carefully prepared data. Begin by gathering clean, relevant data tailored to your specific task and real-world needs. After collection, this data should be tokenized, meaning it’s transformed into a format the model can understand, along with attention masks that guide the model on which parts to prioritize. Organizing the data into batches helps speed up training efficiently.
Using low-quality or unbalanced data can seriously harm your model’s accuracy and ability to perform well on new inputs. This preparation is crucial for building reliable and effective models ready for real-world use.
python
from datasets import load_dataset
from transformers import AutoTokenizer
# Load dataset
dataset = load_dataset("yelp_review_full")
tokenizer = AutoTokenizer.from_pretrained("google-bert/bert-base-cased")
# Tokenize function
def tokenize_function(examples):
return tokenizer(
examples["text"],
padding="max_length",
truncation=True,
max_length=128
)
# Apply tokenization to entire dataset
tokenized_dataset = dataset.map(tokenize_function, batched=True)
# Prepare for training
tokenized_dataset = tokenized_dataset.remove_columns(["text"])
tokenized_dataset = tokenized_dataset.rename_column("label", "labels")
tokenized_dataset.set_format("torch")
The Parameter-Efficient Fine-Tuning Workflow
For organizations aiming to optimize costs and resources, PEFT is a game changer. Here is how it works:
- Pre-training: Begin with a large model trained on diverse, general datasets.
- Task-specific data: Utilize labeled data examples that are relevant to your particular task.
- Parameter identification: Determine which model parameters have the greatest effect on your specific task.
- Subset selection: Choose a small group of parameters to adjust during training.
- Fine-tuning: Update only the chosen parameters while keeping the rest of the model fixed.
- Evaluation: Use validation data to measure performance.
- Iteration: Refine your tuning process based on results.
This approach aligns with LLM training and tuning best practices and supports faster development cycles.
python
import torch
from transformers import Trainer, TrainingArguments
from peft import PeftModel, PeftConfig
# Define training arguments with lower resource requirements
training_args = TrainingArguments(
output_dir="./lora-flan-t5",
learning_rate=1e-4,
per_device_train_batch_size=4,
gradient_accumulation_steps=4,
max_steps=1000,
warmup_steps=100,
logging_steps=50,
save_strategy="steps",
save_steps=200,
evaluation_strategy="steps",
eval_steps=200,
fp16=True, # Use mixed precision
)
# Initialize Trainer with PEFT model
trainer = Trainer(
model=peft_model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset,
)
# Train and save
trainer.train()
trainer.save_model("./lora-flan-t5-final")
# For inference, load the adapter separately
base_model = AutoModelForCausalLM.from_pretrained("google/flan-t5-xxl", load_in_8bit=True)
peft_model = PeftModel.from_pretrained(base_model, "./lora-flan-t5-final")
Tools and Frameworks for LLM Fine-Tuning
Several tools and frameworks facilitate LLM fine-tuning, with Hugging Face’s ecosystem being particularly prominent.
Hugging Face Transformers and Trainer API
One of the most popular toolsets for LLM fine-tuning is from Hugging Face. Their Transformers library includes a powerful feature called the Trainer API, which takes care of most training complexities. This is especially helpful for teams looking to adopt LLM training and tuning best practices without writing code from scratch. The Trainer API allows for easy setup, flexible model customization, and efficient training – even for very large models.
Thousands of companies use Hugging Face tools to fine-tune models for real-world tasks like document classification, customer support chatbots, and product search engines.
Hugging Face’s platform hosts and supports fine-tuning for more than 100,000 models actively.
python
from transformers import TrainingArguments, Trainer, AutoModelForSequenceClassification
from datasets import load_dataset
import evaluate
# Load model and dataset
model = AutoModelForSequenceClassification.from_pretrained("distilbert-base-uncased", num_labels=2)
dataset = load_dataset("glue", "sst2")
tokenized_dataset = tokenize_dataset(dataset) # Custom tokenization function
# Define metrics
metric = evaluate.load("accuracy")
def compute_metrics(eval_pred):
logits, labels = eval_pred
predictions = np.argmax(logits, axis=-1)
return metric.compute(predictions=predictions, references=labels)
# Configure training
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="epoch",
save_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=16,
per_device_eval_batch_size=64,
num_train_epochs=3,
weight_decay=0.01,
load_best_model_at_end=True,
metric_for_best_model="accuracy",
)
# Initialize and train
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_dataset["train"],
eval_dataset=tokenized_dataset["validation"],
compute_metrics=compute_metrics,
)
trainer.train()
Boost Speed with the PEFT Library
To go even further, Hugging Face also offers the PEFT library, which supports parameter-efficient fine-tuning methods like LoRA, Prefix Tuning, and P-Tuning. These methods help reduce computing costs while still delivering strong results – perfect for building production-ready LLMs on a budget.
LoRA, for example, can cut trainable parameters by over 95% while maintaining high accuracy.
Together, these tools support smooth LLM deployment, better LLM performance optimization, and reliable AI model deployment checklists for building custom LLM models that scale.
python
from peft import get_peft_model, LoraConfig, TaskType, PeftModel
# Configure LoRA
lora_config = LoraConfig(
r=16, # rank dimension
lora_alpha=32, # scaling factor
target_modules=["q_proj", "v_proj"], # which modules to apply LoRA to
lora_dropout=0.05,
bias="none",
task_type=TaskType.SEQ_CLS # sequence classification
)
# Create PEFT model
peft_model = get_peft_model(base_model, lora_config)
# After training, merge adapter weights with base model for deployment
merged_model = peft_model.merge_and_unload()
Download the handbook
How CXOs Can Use AI to Maximize Revenue?
By clicking the “Continue” button, you are agreeing to the CrossML Terms of Use and Privacy Policy.
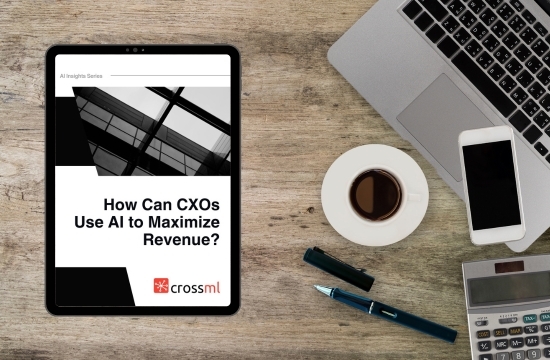
Evaluation and Quality Assurance
Proper evaluation is critical for ensuring your fine-tuned model meets performance requirements before deployment.
- Metrics for Assessing Fine-Tuned Models
The choice of evaluation metrics depends on your specific task:
- For classification: accuracy, F1 score, precision, recall
- For generation: BLEU, ROUGE, perplexity
- For question answering: exact match, F1
Implementing a robust evaluation pipeline:
python
import evaluate
import numpy as np
from transformers import Trainer
# Load metrics
accuracy = evaluate.load("accuracy")
f1 = evaluate.load("f1")
# Define evaluation function
def compute_metrics(eval_pred):
logits, labels = eval_pred
predictions = np.argmax(logits, axis=-1)
return {
"accuracy": accuracy.compute(predictions=predictions, references=labels),
"f1": f1.compute(predictions=predictions, references=labels, average="weighted")
}
# Create evaluation pipeline
evaluator = Trainer(
model=model,
compute_metrics=compute_metrics,
eval_dataset=eval_dataset
)
# Run evaluation
metrics = evaluator.evaluate()
print(metrics)
- Detecting and Addressing Overfitting
Overfitting is a significant risk during fine-tuning, especially with limited data. To detect and mitigate overfitting:
- Monitor training and validation loss curves
- Implement early stopping
- Use techniques like dropout and weight decay
- Consider cross-validation for smaller datasets
python
from transformers import TrainingArguments, EarlyStoppingCallback
# Configure training with early stopping
training_args = TrainingArguments(
output_dir="./results",
evaluation_strategy="steps",
eval_steps=100,
save_strategy="steps",
save_steps=100,
learning_rate=2e-5,
num_train_epochs=10, # Set higher, early stopping will prevent overtraining
weight_decay=0.01, # Regularization to prevent overfitting
load_best_model_at_end=True,
metric_for_best_model="accuracy",
)
# Add early stopping callback
early_stopping_callback = EarlyStoppingCallback(
early_stopping_patience=3, # Stop if no improvement for 3 evaluations
early_stopping_threshold=0.01 # Minimum improvement to count
)
# Initialize trainer with callback
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset,
compute_metrics=compute_metrics,
callbacks=[early_stopping_callback]
)
Production Deployment Considerations
Moving fine-tuned models to production involves several critical considerations.
- Model Serving Infrastructure
Options for serving fine-tuned LLMs include:
- Hugging Face Inference Endpoints: A fully managed service that helps you deploy and scale models easily without handling infrastructure.
- Custom serving solutions: Using frameworks like TorchServe, TensorFlow Serving
Container-based deployment: Packaging models with Docker for Kubernetes environments
- Optimization for Production
Several techniques can improve inference performance:
python
# Quantization for faster inference
from transformers import AutoModelForCausalLM, BitsAndBytesConfig
import torch
# Configure quantization
quantization_config = BitsAndBytesConfig(
load_in_4bit=True,
bnb_4bit_compute_dtype=torch.float16
)
# Load model with quantization
model = AutoModelForCausalLM.from_pretrained(
"your-fine-tuned-model",
quantization_config=quantization_config,
device_map="auto" # Optimize device placement
)
# For PEFT models, load adapter separately
from peft import PeftModel
base_model = AutoModelForCausalLM.from_pretrained(
"base-model",
quantization_config=quantization_config,
device_map="auto"
)
production_model = PeftModel.from_pretrained(base_model, "your-adapter-weights")
Common Pitfalls and How to Avoid Them
Several common issues can undermine fine-tuning efforts. Understanding these pitfalls helps in developing effective mitigation strategies.
- Overfitting: When Your Model Learns Too Much
One big mistake in LLM fine-tuning is overfitting – where your model performs well on training data but poorly on real-world tasks. This usually happens when fine-tuning with small or narrow datasets. It is believed that fine-tuned models often overfit if validation checks are not in place.
To avoid this, use early stopping, dropout layers, and cross-validation techniques. Keep a close eye on metrics like loss and accuracy on unseen validation data during LLM training and tuning.
- Losing Flexibility in Real-World Use
Sometimes, fine-tuning large language models makes them too task-specific, hurting their ability to handle varied or unexpected inputs in production. This affects the success of production-ready LLMs.
To avoid this, use mixed or domain-wide datasets. Multi-task training and regularization techniques help models retain general language skills. Always test your models on multiple test sets with different styles and formats to check for broad understanding.
- Running Out of Compute Power
Large models are expensive to train. LLM performance optimization is key, especially when you are on tight budgets.
Tools like LoRA reduce trainable parameters by 95% while keeping performance high. |
This is a top tactic in modern generative AI fine-tuning strategies and must be on every AI model deployment checklist.
python
# Compare trainable parameters
def count_parameters(model):
total = sum(p.numel() for p in model.parameters())
trainable = sum(p.numel() for p in model.parameters() if p.requires_grad)
print(f"Total parameters: {total:,}")
print(f"Trainable parameters: {trainable:,} ({trainable/total:.2%})")
# Standard fine-tuning (all parameters trainable)
full_model = AutoModelForCausalLM.from_pretrained("llama-7b")
count_parameters(full_model)
# LoRA fine-tuning
lora_config = LoraConfig(r=8, lora_alpha=32, target_modules=["q_proj", "v_proj"])
lora_model = get_peft_model(full_model, lora_config)
count_parameters(lora_model)
Conclusion
LLM fine-tuning is not just about doing little changes to a model. It is a smart, ongoing process that blends performance optimization, resource management, and solid strategy. Techniques like LoRA now make it easier than ever to fine-tune large language models without heavy hardware.
Following LLM fine-tuning best practices like smart data prep, regular evaluation, and layered testing ensures your custom LLM models can scale to production. At CrossML, we help companies build, test, and deploy AI solutions using proven generative AI fine-tuning strategies and a robust AI model deployment checklist.
Success is not about getting it perfect the first time – it is about iterating and refining. With the right tools and partners like CrossML, your team can achieve powerful, scalable, and production-ready LLMs.
FAQs
LLM fine-tuning means customizing a pre-trained large language model using domain-specific data. It helps improve accuracy, relevance, and performance for real-world applications. It is important because it tailors models to business needs, making them smarter, faster, and more efficient for production deployment.
Start with clean, labeled, and diverse datasets relevant to your use case. Select the appropriate model design, implement version tracking, establish baseline results, and set specific performance goals. Tools like Hugging Face and CrossML’s setup frameworks simplify this process for enterprise-grade tuning.
Fine-tuning boosts accuracy, reduces inference time, and enables domain adaptation. It supports building custom LLM models tailored to your tasks, improves LLM performance optimization, and helps reduce compute costs using parameter-efficient methods like LoRA and PEFT.
Fine-tune your LLM when off-the-shelf models do not meet your domain needs or when improved performance, accuracy, or efficiency is required. It is ideal before full-scale LLM deployment to ensure your model delivers value under production workloads.
Challenges include overfitting, data bias, high compute costs, and loss of generalization. If LLM fine-tuning is not done properly, the results can become inconsistent or unreliable. Mitigate risks with cross-validation, PEFT techniques, and help from experienced partners like CrossML.